I have a color wheel for my game, and I get an hsv value from it, then using ‘in engine’ api, I can get an rgb from the hsv values (the rgb ranges 0-1 for its color values)
However, I need to do the opposite. I need to start with an rgb, and convert that to an hsv, and I know the v is just a position on a slider, but how do I calculate where to put the color wheel marker based on the h, and s value.
here is the color wheel I am using.
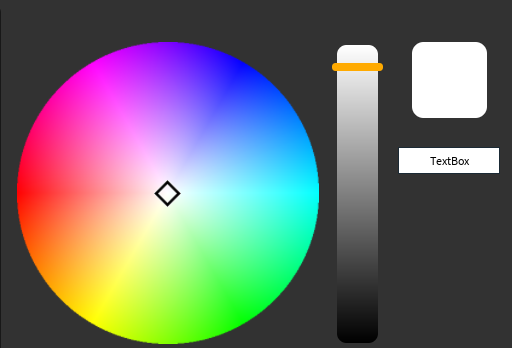
I appreciate any help on this, thanks
Edit, I forgot to add, I am using lua, in the Roblox engine.
<code>
local function GetColor()
local wheelCenter = Vector2.new(
Wheel.AbsolutePosition.X + (Wheel.AbsoluteSize.X/2),
Wheel.AbsolutePosition.Y + (Wheel.AbsoluteSize.Y/2))
local markerCenter = Vector2.new(
WheelMarker.AbsolutePosition.X + (WheelMarker.AbsoluteSize.X/2),
WheelMarker.AbsolutePosition.Y + (WheelMarker.AbsoluteSize.Y/2))
local h = (math.pi - math.atan2(markerCenter.Y - wheelCenter.Y, markerCenter.X - wheelCenter.X)) / (math.pi * 2)
local s = (wheelCenter - markerCenter).Magnitude / (Wheel.AbsoluteSize.X/2)
Slider.UIGradient.Color = ColorSequence.new{
ColorSequenceKeypoint.new(0, Color3.fromHSV(math.clamp(h, 0, 1), math.clamp(s, 0, 1), 1)),
ColorSequenceKeypoint.new(1, Color3.new(0, 0, 0))
}
local color = Color3.fromHSV(math.clamp(h, 0, 1), math.clamp(s, 0, 1), math.clamp(Value, 0, 1))
Swatch.BackgroundColor3 = color
Result.Value = color
end
--=======================================================
function WheelMath(input)
local r = Wheel.AbsoluteSize.x/2
local d = Vector2.new(input.Position.x, input.Position.y) - Wheel.AbsolutePosition - Wheel.AbsoluteSize/2
if (d:Dot(d) > r*r) then
d = d.unit * r
end
WheelMarker.Position = UDim2.new(.5, d.x, .5, d.y)
GetColor()
end
</code>